MAY 6 → MAY 9 – 2024
Tasks
Technical Developments
- Implementing paper collection hint
- As we found that Rive (an application for vector animations that Yonah & Joris were using) did not work in our Unity project, we had to come up with a different solution.
- I decided to write a script that would go through sprites and replace the image with the corresponding sprite.
(see code below)
- Working on paper physics
- As the interactions for scene three were finished, we decided to get started on the next-most interactions heavy one. This would be the garden, as there was an interaction with paper flying around.
- I researched paper physics and tried to find resources online of people who had previously tackled this issue.
Miscellaneous
- Ascension day
Double Diamond
My main objective this week was tackling the issue that falling paper would be. The physics are really hard to replicate in a simple manner as it would also have to be performant enough for Android.
Discover
I conducted research into paper physics and tried to find resources of people that had previously done work like this. I also researched what would be the best way to create the effect.
Define
Eventually I decided on just animating gameobjects through script, some sort of visual effect would have been possible too but based on the particle systems in scene three I decided against it with perfomance in mind.
The goal would be to write a script that can be activated from a ‘Controller’ after which the paper flies up and floats down similarly to an actual piece of paper.
Develop
Knowing my goal I got to work and quickly stumbled upon a resource on GitHub (listed below) that was called FeatherFall. Which was a script for Unity that closely replicated a piece of paper or other light object falling down.
After importing and tweaking this script a bit I also wrote my own Paper script, that would inherit from Interactable and would be called by my InputManager when interacted with. This followed the same structure as previously used in scene three for the trash. Next to that I wrote a controller script that could simultaneously call all paper sheets.
Deliver
The progress for this week was recorded in a video. Which was also sent to our client, after which he provided feedback about the paper not being allowed to the touch the ground as it was holy. I then explained to him what the technical ramifications would be and we decided it would be fine in this case.
Plan
The plan for next week is to finish the second scene including paper interaction and physics, implement new voice overs that our client sent us. Also, I will be looking for a way to control emotions for our characters as 3D modelling and rigging them does not seem feasible anymore at this moment in time.
Research
Most of the research I have done this week was aimed towards figuring out how paper functions in terms of physics and how to convert that into Unity. For this I watched the following videos.
Falling Paper VFX for Unity https://www.youtube.com/watch?v=D0rzbu5XHOk
[Unity Asset] Falling Paper VFX https://www.youtube.com/watch?v=GDoSP_5rRBg
I also found a great resource on GitHub and read some forum posts.
How to make a simple object fall as a piece of paper? https://discussions.unity.com/t/how-to-make-simple-object-that-would-fall-as-piece-of-paper/252318
VFX Flying Paper https://assetstore.unity.com/packages/vfx/particles/vfx-flying-paper-95726
unity-feather-fall https://github.com/bjennings76/unity-feather-fall
Reflection
The paper physics were very difficult to pin down but I still feel like I could have had it done faster instead of having to move it to next week. Other than that, I am quite satisfied with my current progress; The second scene is coming along nicely due to the structure I had already set up weeks ago, as well as coming up with a creative solution for the animations that weren’t possible in rive.
Media & code
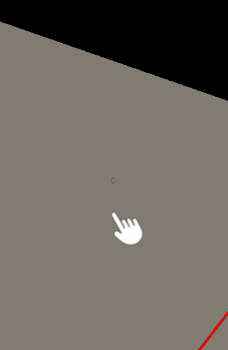
public class PaperController : MonoBehaviour1
{
[SerializeField] private List<PaperSheet> papers;
public void BlowPapers()
{
foreach(PaperSheet paper in papers)
{
paper.Blow();
}
}
}
public class ImageAnimation : MonoBehaviour
{
public Sprite[] sprites;
public int spritePerFrame = 6;
public bool loop = true;
public bool destroyOnEnd = false;
private int index = 0;
private Image image;
private int frame = 0;
private bool isPlaying = false;
protected void Awake()
{
image = GetComponent<Image>();
}
protected void OnEnable()
{
isPlaying = true;
}
protected void OnDisable()
{
isPlaying = false;
}
protected void Update()
{
if (isPlaying)
{
if (!loop && index == sprites.Length) return;
frame++;
if (frame < spritePerFrame) return;
image.sprite = sprites[index];
frame = 0;
index++;
if (index >= sprites.Length)
{
if (loop) index = 0;
if (destroyOnEnd) Destroy(gameObject);
}
}
}
}2